Laravel
Laravel [1] is a PHP framework using elements from other tools such as Symfony 2 [2] and Bootstrap [3]. The documentation on this framework can be found on multiple places on the Internet.
But to use Laravel on an OS/X Mac in combination with MAMP-PRO may cause some difficulties.
Artisan
Artisan is the command line tool for Laravel can be used for:
- Generating a boilerplate controller
i.e. $ php artisan controller:make user - Managing database with migrations
i.e. $ php artisan migrate:install - Filling the database with basic data for testing via database seeds
i.e. $ PHP artisan db:seed - Running unit tests
i.e. $ php artisan test - Routing
- Configuring an application
- Creating new artisan commands
- Creating a new workbench package
- Maintenance
i.e. $ php artisan down
When trying to run the command line tools of Laravel [4] I got an error:
$ php artisan Mcrypt php extension required. ...
The problem is caused by the fact that the used version of PHP is not the correct one. First try:
$ php -v PHP 5.4.30 (cli) (built: Jul 29 2014 23:43:29) Copyright (c) 1997-2014 The PHP Group Zend Engine v2.4.0, Copyright (c) 1998-2014 Zend Technologies with Xdebug v2.3.0dev, Copyright (c) 2002-2013, by Derick Rethans
Now look in the Application directory of MAMP
$ la /Applications/MAMP/bin/php total 0 drwxrwxr-x 6 HaFrMpro admin 204 Jan 22 2013 php5.2.17 drwxrwxr-x 6 HaFrMpro admin 204 Jan 22 2013 php5.3.20 drwxrwxr-x 7 HaFrMpro admin 238 Jan 22 2013 php5.4.10
Choose the PHP version 5.4.10 and place the following code in you .bash_profile
export MAMP_PHP=/Applications/MAMP/bin/php/php5.4.10/bin export PATH="$MAMP_PHP:$PATH"
Let me know if this helps
Installing with composer
If you have Composer installed globally, installing the Laravel installer tool is as simple as running the following command:
composer global require "laravel/installer"
Once you have the Laravel installer tool installed, spinning up a new Laravel project is simple. Just run this command from your command line:
laravel new projectName
This will create a new subdirectory of your current directory named {projectName} and install a bare Laravel project in it.
MAMP PRO
Laraval runs easily on Mac.
- Requirements:
- Installed MAMP/MAMP Pro.
- Install Laravel using composer
- Use the link https://laravel.com/docs/9.x/installation
- Create new Host in MAMP Pro and Points the Document Root to
- The Public folder of the Laravel installation.
- Save and restart Apache MySQL and Optional your other Mamp Pro Services.
The one big disadvantage of Laravel is you can not use it on a Shared Hosting Server [5].
See the following example issues:
However articles on the Laravel Forum/Community show examples on how to do it [6][7]
Cron Jobs
If you are using a shared hosting service, you will find scheduling Cron Jobs extremely difficult. This holds for Laravel-based applications as well, as you will be unable to set task scheduling functionality on shared hosting websites.
SSH or Command Terminal
You must know that most shared hosting service providers don’t offer SSH Terminal features.
Also, there is no version control support available, hence, being a developer, you have limited options to work with.
You can work with FTP only.
Most of the developers remain highly critical and discourage the deployment of the Laravel app via FTP.
Moreover, if there is no SSH Terminal available, you won’t have any access to the composer, artisan, or any other command-line tools to work with.
Magic of Laravel
Laravel's magic works:
- Laravel has a lot of core components
- When any request hits the Laravel framework, it bootstraps and loads its Application class
- Laravel's Application class extends the container class, so the container class is also loaded
- Framework loads and executes the register method of all the service providers that are in the providers array at app/config/app.php
- Each service provider by the Laravel packages has a registered method that binds each of the package classes with the IoC container
- Once each package is bound with the IoC container, you can use packages such as Auth, Cache, and Log in your application
Packages are the easiest way to add third party code in Laravel [8].
Laravel
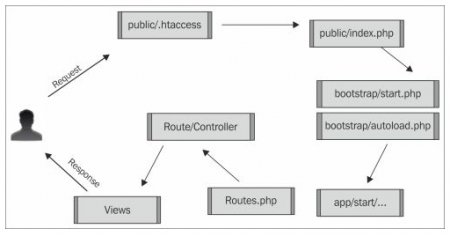
Request Life Cycle
High level overview of Laravel [9]:
- Request enters public/index.php file (i.e. blog/public/index.php).
- The bootstrap/start.php file (i.e. /blog/bootstrap/start.php) creates Application and detects environment.
- Internal framework/start.php file (i.e. blog/vendor/laravel/framework/src/Illuminate/Foundation/start.php ) configures settings and loads service providers.
- Application app/start files (i.e. blog/app/start/) are loaded.
- Application app/routes.php file (i.e. blog/app/routes.php) is loaded.
- Request object sent to Application, which returns Response object.
- Response object sent back to client.
Start Files
Application's start files are stored at app/start (i.e. blog/app/start/). By default, three are included with your application:
- global.php,
- local.php,
- artisan.php
Application Events
Do pre and post request processing by registering
- before,
- after,
- finish,
- shutdown
application events:
App::before(function($request) { // }); App::after(function($request, $response) { // });
Routing
Most of the routes for your application will be defined in the app/routes.php file [10]. The simplest Laravel routes consist of a URI and a Closure callback.
- Basic Routing
- Route Parameters
- Route Filters
- Named Routes
- Route Groups
- Sub-Domain Routing
- Route Prefixing
- Route Model Binding
- Throwing 404 Errors
- Routing To Controllers
Basic GET Route
Route::get('/', function() { return 'Hello World'; });
Basic POST Route
Route::post('foo/bar', function() { return 'Hello World'; });
Registering A Route For Multiple Verbs
Route::match(array('GET', 'POST'), '/', function() { return 'Hello World'; });
Registering A Route Responding To Any HTTP Verb
Route::any('foo', function() { return 'Hello World'; });
Forcing A Route To Be Served Over HTTPS
Route::get('foo', array('https', function() { return 'Must be over HTTPS'; }));
Often, you will need to generate URLs to your routes, you may do so using the URL::to method:
$url = URL::to('foo');
Requests & Input
The request & Input consists on [11]:
- Basic Input
- Cookies
- Old Input
- Files
- Request Information
Views & Responses
Consists on [12]:
- Basic Responses
- Redirects
- Views
- View Composers
- Special Responses
- Response Macros
Controllers
Consists on [13]:
- Basic Controllers
- Controller Filters
- Implicit Controllers
- RESTful Resource Controllers
- Handling Missing Methods
Errors & Logging
The logging and error consists on [14]:
- Configuration
- Handling Errors
- HTTP Exceptions
- Handling 404 Errors
- Logging
See also
General
- Twitter, Official Twitter page
- Learning Laravel, Official Webpage
- Tuts+Premium, A good place to learn Laravel
- Laravel Knowledge Base, Knowledge base
- Laravel ScreenCasts by Jeffrey Way.
- Laravel Snippets, Laravel snippets collected by John Kevin Basco
- Tutplus, Nettus+, Great web development blog with lots of Laravel articles.
Blogs
- Blog Elena Kolevska, Adding extra Validators.
Books
- ASIN: B07Q3T513R: Laravel: Up & Running, A Framework for Building Modern PHP Apps, (English Edition), Matt Stauffer, is a developer and a teacher. He is a partner and technical director at Tighten Co., blogs at mattstauffer.co, and hosts The Five-Minute Geek Show and the Laravel Podcast.
Documentation
- Laravel Artisan, Complete Artisan Cheatsheet
- Laravel Docs, Documentation main
- Laracast, Push your web development skills to the next level, through expert screencasts on Laravel, Vue, and so much more.
- Laravel Docs, API 4.2
- Laravel CheatSheet, by Jesse O'Brien
- Laravel News, News from/on Laravel
Internal
- Bootstrap, Bootstrap is (the most | a very) popular HTML, CSS, and JS framework for developing responsive, mobile first projects on the web.
Tutorials
- Codecondo.com, Laravel news reources and tutorials. Top 13 Places to Visit for Laravel Tutorials, Resources & News.
- Laravel.io, Forum, Community.
Laravel does sport a forum community, which is great as it’s very populated and there are plenty of people in the community who will be more than happy to help you. You can find some great packages and resources built by people who love coding and creating with Laravel. - Reddit.com, Laravel on reddit, Tons of resources and questions answered already. Make sure to search before asking, but feel free to help others as well, it’s always appreciated.
- TutsPlus, Nettus+, Laravel. See also above
- LaraShout, Free tutorial.
Samples
- RBAC Laravel, Tutorial implementing a Role Based Access Control in Laravel.
Reference
- ↑ laravel.com, Laravel's Home page.
- ↑ Symfony SensioLabs Symphony Homepage. Symphony is a set of reusable {HP components.
- ↑ Bootstrap Homepage.
Bootstrap is the most popular HTML, CSS, and JS framework for developing responsive, mobile first projects on the web. - ↑ Alecadd, How to solve the mcrypt php extension required problem.
- ↑ Cloudways.com, Stay away from Laravel Shared Hosting.
- ↑ Laravel Community, Deploying Laravel 7 on shared hosting (Hostinger-1).
- ↑ laravel article, Deplot Laravel on shared host.
- ↑ Packagist.org, List of Packages available for Laravel.
- ↑ Laravel Docs, Request Life Cylce.
- ↑ Laraval Docs, Routing.
- ↑ Laravel Docs, Requests
- ↑ Laravel Docs, Responses.
- ↑ Laravel Docs, Controllers
- ↑ Laravel Docs, Logging and Errors.